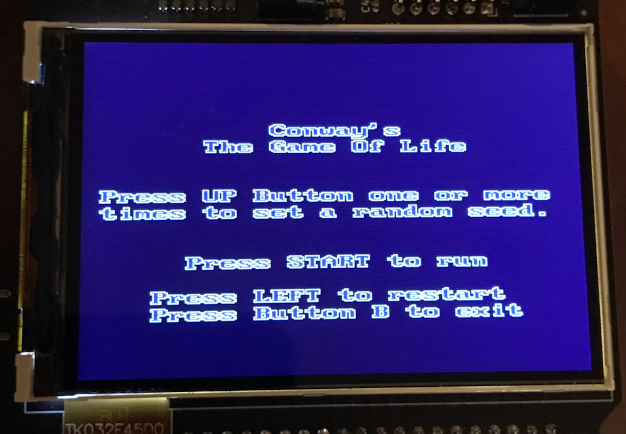
As a project for me to work on I decided to try coding in C Conway’s Game Of Life on the SuperCon Badge. What a hoot and adventure. Every time I thought I was about finished I would think of adding something else and end up breaking something different.
Well, it wasn’t that bad. I really hadn’t programmed in a variant of C like this in a while. ANDs and ORs were not cooperating so I separated them out into their own IF statements. The code began to look ugly, but it worked.
I thought I was finally done and I decided to add a restart button. I ended up writing a little demo program to make sure I had the coding of the buttons correct. All of the examples for checking on button presses included this little bit of code that could cause a delay. Whomever came up with the default code examples for the badge included the “inefficient” delay. Hey, it got the job done.
Once I had the buttons example running I decided to try to get rid of the “inefficient” delay. To get the program running at about the desired speed I had the little section in the MAIN loop running once every 50,000 times. This is a fast little chip on the badge.
I haven’t taken out the “inefficient” delay loop out of the Game Of Life program, yet, but I am ready to document the story so far. After I got those pictures loaded into the computer and looked at the screen shot of the badge I see a change I’d like to make. I think I need to add a line above the bottom two lines that should say, “While running press:”. This way the person will know that B to exit and LEFT to reset will only happen once the program is running.
There are lots of web pages describing Conway’s Game of Life. Some just give the premise and others offer code snippets. As I understand it, for every space on the screen, if there is already a dot in place in that space then you would look at the eight adjoining, or touching, spaces around the space in question. Add up all of the dots around the space in question. If there are two or three dots the dot in that original space will continue to be a dot. If there are zero or one dots in all the surrounding spaces then the original dot will cease to be a dot and will become an empty space due to starvation. Like wise, if there are four or more dots in total around the original space with a dot that original dot will also cease to exist due to overcrowding. The original spaces that you would check that are in a corner or on the edge of the playing field are at a disadvantage due to less number of spaces surrounding them. You check every space within the playing field before you refresh the screen. I used a second array to hold the results and then sent that to the display.
A second thing to consider is if the space you are checking starts out empty it can become populated with a dot if there are three, and only three, spaces around that original space with a dot in them.
(I just thought that I could have the screen wrap around from bottom to top and left to right. Programming for another day.)
The ‘game’ runs until the screen is empty or certain patterns end up on the screen. A 2×2 block of dots will just sit there, for example. A vertical straight line of three will switch to a horizontal straight line of three and back again.
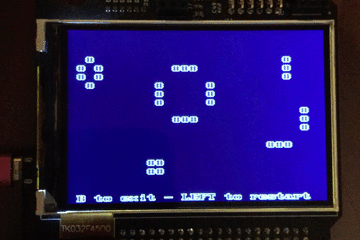
At one point I thought I was done with the game. It was really boring because it always had the same result. I decided to put in a way to give a random seed into the mix. That shook things up a bit.
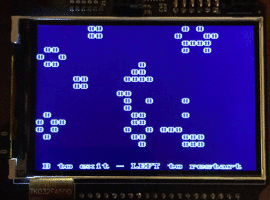
At another point when I was checking how other programs were running on the badge I noticed Badgetris turned off those annoying LEDs. They are quite bright and bounce off of my glasses when I am looking at the screen. I included that line from the Badgetris code into my program.
Should I be including code snippets as I go along? Perhaps…
I am going to have to go through the code and add comments so you will know what I am doing.
There was one part that particularly did not work as I was coding. I could not figure out why it would not work. I thought I should be able to use
if (playArea[29][18] == 1 && (holder == 2 || holder ==3)) {
newPlayArea[29][18] = 1;
} else { newPlayArea[29][18] = 0; }
// now check holder status
if (playArea[29][18] == 0) {
if (holder == 3) {
newPlayArea[29][18] = 1;
} else { newPlayArea[29][18] = 0; }
}
The top part with the AND and OR never went into that area. The screen cleared quickly and the game was over shortly. Splitting the AND and Ors up really helped. But, I think it shouldn’t.
if (playArea[29][18] == 1) {
if (holder == 2) {
newPlayArea[29][18] = 1;
} else if (holder == 3) {
newPlayArea[29][18] = 1;
} else {
newPlayArea[29][18] = 0; } }
// now check holder status
if (playArea[29][18] == 0) {
if (holder == 3) {
newPlayArea[29][18] = 1;
} else { newPlayArea[29][18] = 0; }
}
In actuality I think I had the OR before the AND.
I also had noticed the error in “if(playArea[29][19] = 0;}}”. The error is the 19 should be should be 18.
if ((holder == 2 || holder ==3) && playArea[29][18] == 1){
In my pea little brain, this should work, but it didn’t. Perhaps I should try the type of
if ((playArea[29][18] == 1) && ((holder == 2) || (holder ==3)))
That just might work with all those extra parantheses. And the OR part is second instead of being checked first as in my original attempt. I’ll go try it and come back with results.
I tried many variations of having three comparisons inside the IF statement. Perhaps I am doing it wrong.
if (((holder == 2) || (holder == 3)) && (playArea[xx][yy] == 1)) {
if ((playArea[xx][yy] == 1) && ((holder == 2) || (holder == 3))) {
and even
if ((playArea[xx][yy] == 1) && (holder == 2 || holder == 3)) {
None of the checking three booleans inline worked so I am breaking them all out. It is not that it matters, but smaller code will run a bit quicker.
While I was testing the code I still find that I don’t like the difficult readability of the default text on the screen. I also thought putting a background on the play area would be fun. This could become a never ending project. I don’t want that. 😀
–++–
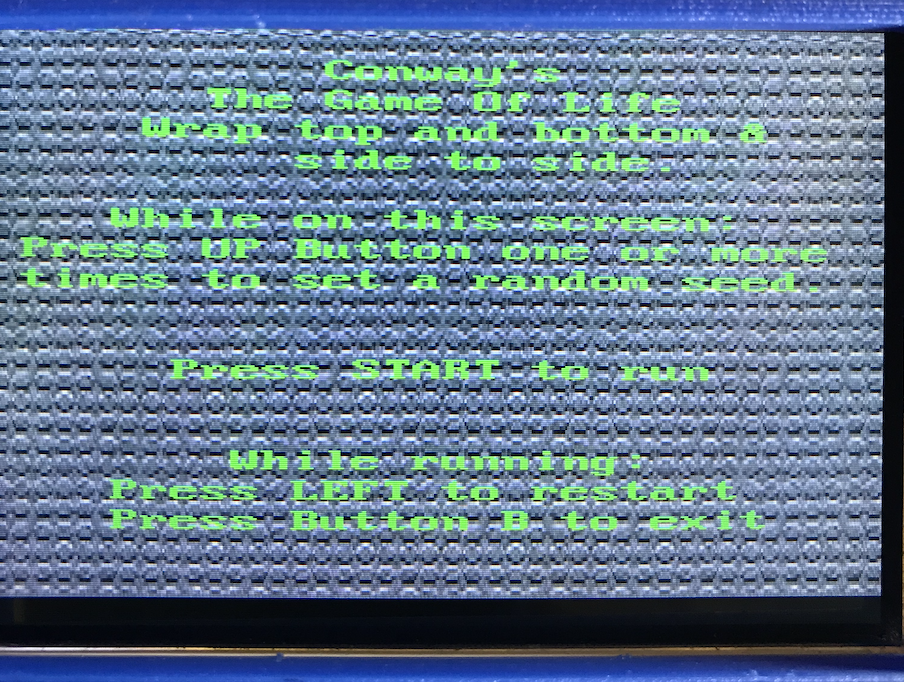
I have tossed in a background picture. Now it is harder to see the game. I’ll have to create a different character in a tile map and use that as the character. The default blue character with a white outline is blurry looking anyway. I am looking at it with eyes that have over six decades of use.
I changed the tileset so that I can change the color of the font. That was pretty easy to do not that I know that the graphic files should only have 16 colors. In GIMP it is easy to set the colors to indexed and 16 colors. Then you can play with the index so you have the color you want.
I guess I better change the pictures so they match what the screen looks like now.
—+++—
Well, riding in the car back from San Diego while my wife was driving I decided to work on a version of GOL that uses wrapping on the screen. There is now a version that wraps between the top and bottom as well as side to side. The results are different. It was easy to add the code.
I wonder if I can add the code as an option on the main screen. Bet I can, if I want to do that. You can do anything if you put your mind to it.
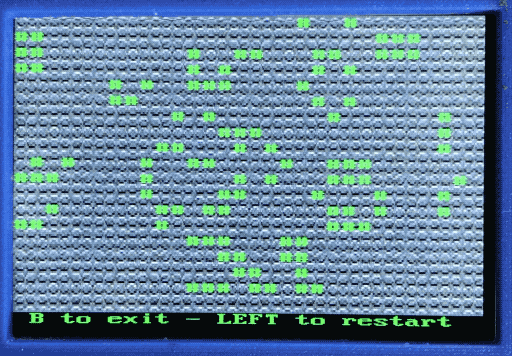
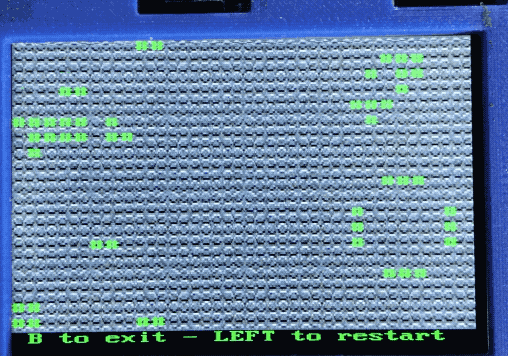
I still need to post pictures of the game running with a background and green hash characters.
—+++—
I have uploaded files to GitHub for both versions the Game Of Life. There is the version that does not wrap around the screen edges and one that does.
https://github.com/gbushta/GameOfLife
https://github.com/gbushta/Game-Of-Life-2
The first link is the one that wraps around the screen. It creates an .elf file that is GOL4. The .elf file is also available so you don’t have to compile the program before copying it to your Hackaday Supercon Badge. There is also a .elf file for GOL2, which is the one that does not wrap around the edges.